할머니의 콤퓨타 도전기
Axios 본문
Axios
- Axios는 브라우저, Node.js를 위한 Promise API를 활용하는 HTTP 비동기 통신 라이브러리
- 브라우저 환경: XMLHttpRequests 요청 생성
- Node.js 환경: http 요청 생성
- 백엔드랑 프론트엔드 통신을 쉽게 하기 위해 Ajax와 더불어 사용
- Promise(ES6) API 지원
- 요청/응답 차단
- 요청/응답 데이터 변환
- HTTP 요청 취소
- HTTP 요청과 응답을 JSON 형태로 자동 변환
HTTP
developer.mozilla.org/ko/docs/Web/HTTP
HTTP | MDN
하이퍼텍스트 전송 프로토콜(HTTP)은 HTML과 같은 하이퍼미디어 문서를 전송하기위한 애플리케이션 레이어 프로토콜입니다. 웹 브라우저와 웹 서버간의 커뮤니케이션을위해 디자인되었지만, 다
developer.mozilla.org
브라우저 호환성
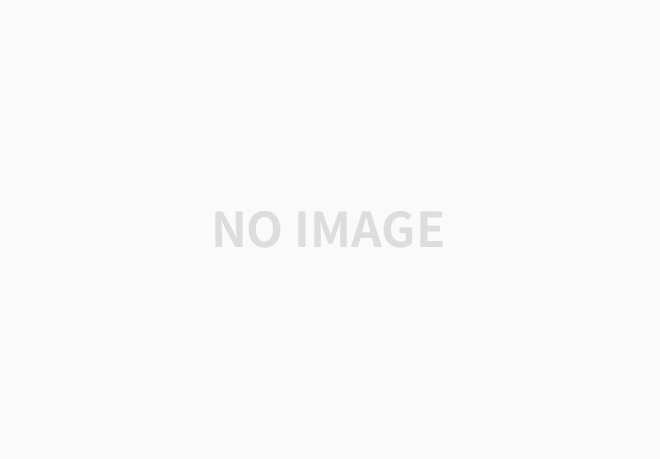
Axios 설치
- npm i axios
- import axios from 'axios'
HTTP Methods
- 클라이언트가 웹 서버에게 사용자 요청의 목적/종류를 알리는 수단
- HTTP Methods 중 Axios 통신하는데 사용하는 메서드
- GET
- 입력한 url에 존재하는 자원에 요청
- 서버에게 어떤 데이터를 가져오는 용도
- GET 메서드는 값이나 상태 등을 바꿀 수 없다
axios.get(url, [,config])
import axios from 'axios';
axios.get('https://yts.mx/api/v2/list_movies.json').then((response)=>{
console.log(resposne.data);
}).catch((error)=>{
console.log(error);
})
- POST
- 새로운 리소스를 생성할 때 사용
- POST Method의 두 번째 인자는 본문으로 보낼 데이터를 설정한 객체 리터럴을 전달
- 로그인, 회원가입 등 사용자가 생성한 파일을 서버에다가 업로드할 때 사용
- Post를 사용하면 주소창에 쿼리스트링이 남지 않기 때문에 GET보다 안전
axios.post('url',{
data 객체
}, [,config])
- DELETE
- REST 기반 API 프로그램에서 데이터베이스에 저장되어 있는 내용을 삭제하는 목적으로 사용
- DELETE Method는 HTML Form 태그에서 기본적으로 지원하는 HTTP 메서드는 아님
- DELETE Method는 서버에 있는 데이터베이스의 내용을 삭제하는 것이 주 목적
axios.delete(url, [,config]);
axios.delete('/example/list/30').then(function(response){
console.log(response);
}).catch(function(err){
throw new Error(err);
})
- PUT
- REST 기반 API 프로그램에서 데이터베이스에 저장되어 있는 내용을 갱신하는 목적으로 사용
- PUT Method는 HTML Form 태그에서 기본적으로 지원하는 HTTP 메서드는 아님
- 서버에 있는 데이터베이스의 내용을 변경하는 것이 주 목적
axios.put(url, [, data[, config]])
Axios 서버 통신
- 백엔드 없이 서버 통신을 하기 위해 FakeServer를 사용했다
Reqres - A hosted REST-API ready to respond to your AJAX requests
Native JavaScript If you've already got your own application entities, ie. "products", you can send them in the endpoint URL, like so: var xhr = new XMLHttpRequest(); xhr.open("GET", "https://reqres.in/api/products/3", true); xhr.onload = function(){ conso
reqres.in
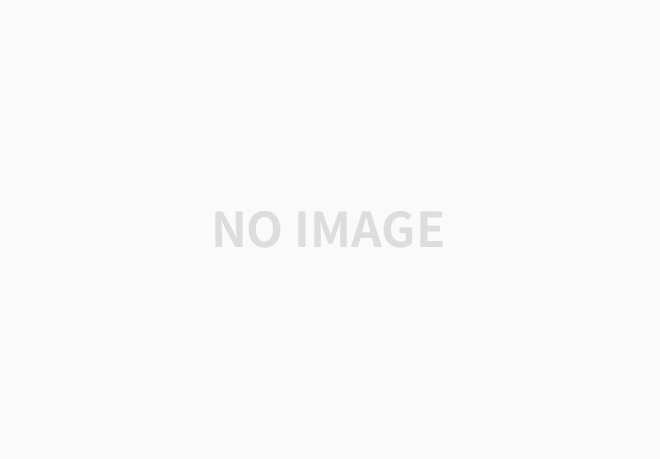
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>JS Bin</title>
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
</head>
<body>
<div>
<input type="email" placeholder="input your email" id="email" value="">
</div>
<div>
<input type="password" placeholder="input your password" id="password" value="">
</div>
<input type="button" onclick='onLogin()' value="LOGIN">
</body>
<script>
function onLogin() {
const email = document.getElementById('email');
const password = document.getElementById('password');
axios({
method: 'POST',
url: 'https://reqres.in/api/login',
data: {
"email": email.value,
"password": password.value
}
}).then((res) => {
console.log(res);
}).catch((error) => {
console.log(error);
throw new Error(error);
})
}
</script>
</html>
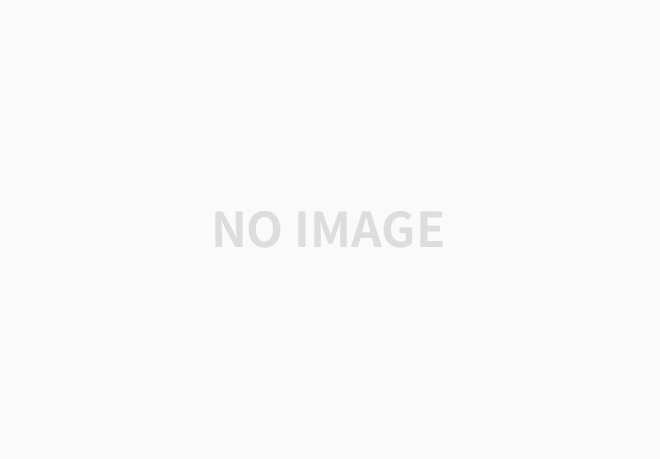
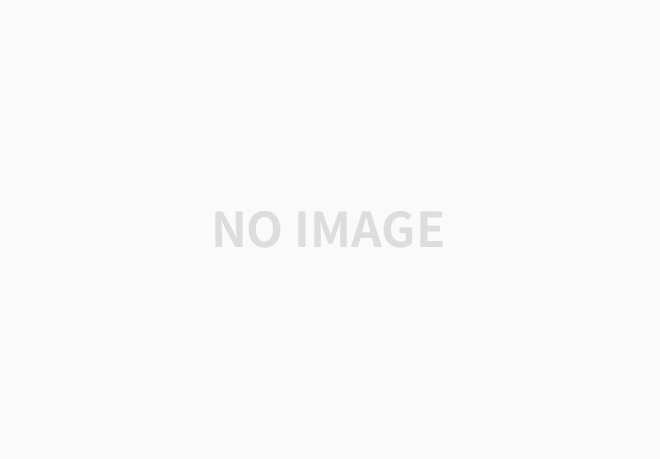
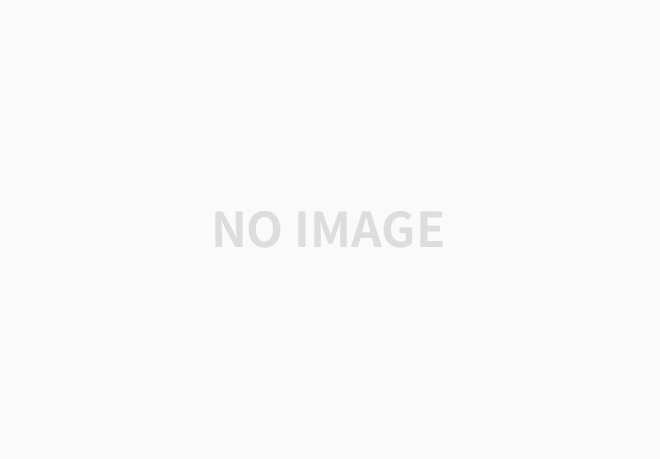
Axios 관련 내용을 찾다가 정리가 잘 되어있는 곳을 발견했다. 이 사이트를 읽어보는게 좋을 것 같다. ✨
이듬.run/axios/guide/#axios%EB%9E%80
Axios | Axios 러닝 가이드
xn--xy1bk56a.run
참고
'웹 개발 용어 및 개념 정리' 카테고리의 다른 글
CDN (Content Delivery Network) (0) | 2022.01.19 |
---|---|
정규 표현식 정리 (0) | 2021.05.15 |
Rendering Engine working process (0) | 2021.04.30 |
HTTP Protocol (+HTTPS) (0) | 2021.04.30 |
Browser rendering process (0) | 2021.04.29 |